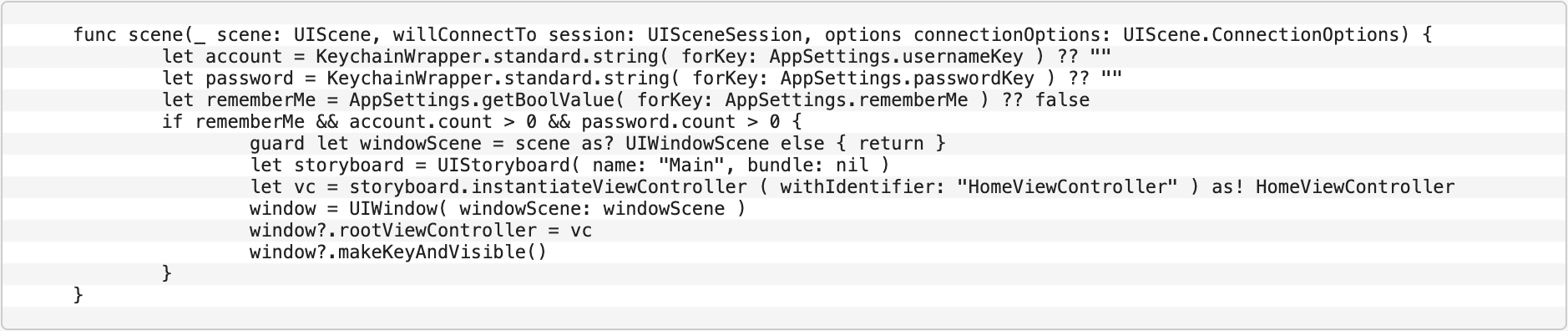
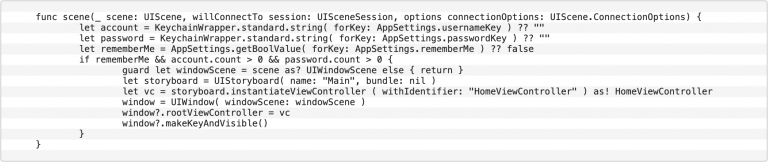
I had trouble getting this to work. Actually, I had no trouble getting it to work – I had trouble finding the necessary information online (documentation) to tell me how to do this. This is one of those things where I’m never sure how the first person to do it figured it out. Maybe I could have figured it out but since I switch between platforms and programming languages almost daily, it’s hard to become a real expert at any of this.
Here is the code in the SceneDelegate class.
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) {
let account = KeychainWrapper.standard.string( forKey: AppSettings.usernameKey ) ?? ""
let password = KeychainWrapper.standard.string( forKey: AppSettings.passwordKey ) ?? ""
let rememberMe = AppSettings.getBoolValue( forKey: AppSettings.rememberMe ) ?? false
if rememberMe && account.count > 0 && password.count > 0 {
guard let windowScene = scene as? UIWindowScene else { return }
let storyboard = UIStoryboard( name: "Main", bundle: nil )
let vc = storyboard.instantiateViewController ( withIdentifier: "HomeViewController" ) as! HomeViewController
window = UIWindow( windowScene: windowScene )
window?.rootViewController = vc
window?.makeKeyAndVisible()
}
}
That works!
I do see a change I need to make, now that I’m looking closer at this. The “guard … else { return }” statements should not exist. A guard is good at the beginning of a function but it not at all appropriate inside of a bunch of code like this. The problem is that someone might not see it and add code after this if… block. Then their code won’t run if that fails, even though the app should fall through to working with the default view controller. There’s no reason to completely fail if that guard fails.